Visual Studio Code is a capable editor for node.js and JavaScript applications. In fact, Visual Studio Code itself is written is JavaScript! Being cross platform, it's great for ensuring a consistent development experience across teams that work on multiple operating systems including Windows, macOS, and Linux. Visual Studio Code (VS Code) is a code editor made by Microsoft that is used by developers worldwide due to the many tools and features it offers. Its features can be further enhanced by the use of extensions. VS Code can also be used to debug many languages like Python, JavaScript, etc. And has made debugging Node.js apps a very simple.
Working on a coding project inside of Visual Studio is hugely helpful. VS does so many things to support the developer, that I myself (and millions of others) define it as indispensable. Whether I’m writing C# or JavaScript, I still want to do it in Visual Studio.
I have a lot of Node.js code on my box and occasionally I’ll get into a situation when I have an existing Node.js project (one that I didn’t create initially using Visual Studio), and I want to open it in VS.
When you create a new Node.js project in Visual Studio, it gives you the basic files - app.js, package.json, and README.md - and then it gives you a .njsproj file. That’s the file that lights VS up with all of the additional help that’s specific to Node.js. That’s the file we essentially need to create in an existing project, but how? It’s not exactly a new project, but according to Visual Studio’s definition, it’s not an existing project either.
I’ll show you how. But first, you have to have Visual Studio (I’m running the free Visual Studio Community 2013 with Update 4) and the Node.js Tools for Visual Studio.
First, open Visual Studio and choose File | New Project…
Under the Templates section you should be able to expand a JavaScript section and then choose Node.js.
On the right, you will see a big list of project templates, and notice the second one (it’s the second on my list at least) - From Existing Node.js code. That’s the one. Hit it.
The values in the 3 boxes on the lower end of the box - Name, Location, and Solution Name - by the way, are used to populate default values on subsequent screens, but you’ll have a chance to change them. I recommend you put the name of your project in the Name field and the path to it in the Location.
Then hit OK.
The first wizard screen prompts you for the location of your existing Node.js project. If you populated the Location field on the New Project screen, then this will already be set. The rest of this screen is self-explanatory.
The wizard next guesses what is the entry point. If it’s not right then point it to the right one.
Finally, you need to indicate the name of the .njsproj file, and again it’s going to pull a default from the New Project screen if you filled that out.
And there you have it. You’ve essentially gotten a .njsproj file created where it should be. If you created this new project from the File menu like I did in my example, then your new Node.js project is inside of an unsaved solution file, so when you close Visual Studio or the solution, it’s going to prompt you to save it. The other approach - if you already had a solution - would be to open it, right click the solution file, and then choose New Project from the context menu.
Have fun with Node.js!
In this guide we’ll cover how to set up your Node.js development environment for an Express project. We’ll also walk through some helpful tools that we recommend for all Node.js applications that use Twilio: ngrok and the Twilio Node.js SDK
Install Node.js
How you install Node.js varies depending on your operating system.
Operating System | Instructions |
---|---|
OS X | The easiest way to install Node.js on OS X is to use the official installer from nodejs.org. You can also use Homebrew if you prefer. |
Windows | The easiest way to install Node.js on Windows is the official installer from nodejs.org. You can also use Chocolatey if you prefer. |
Linux | The exact instructions to install Node.js vary by distribution. Find instructions for yours here. |
Install a Text Editor or IDE
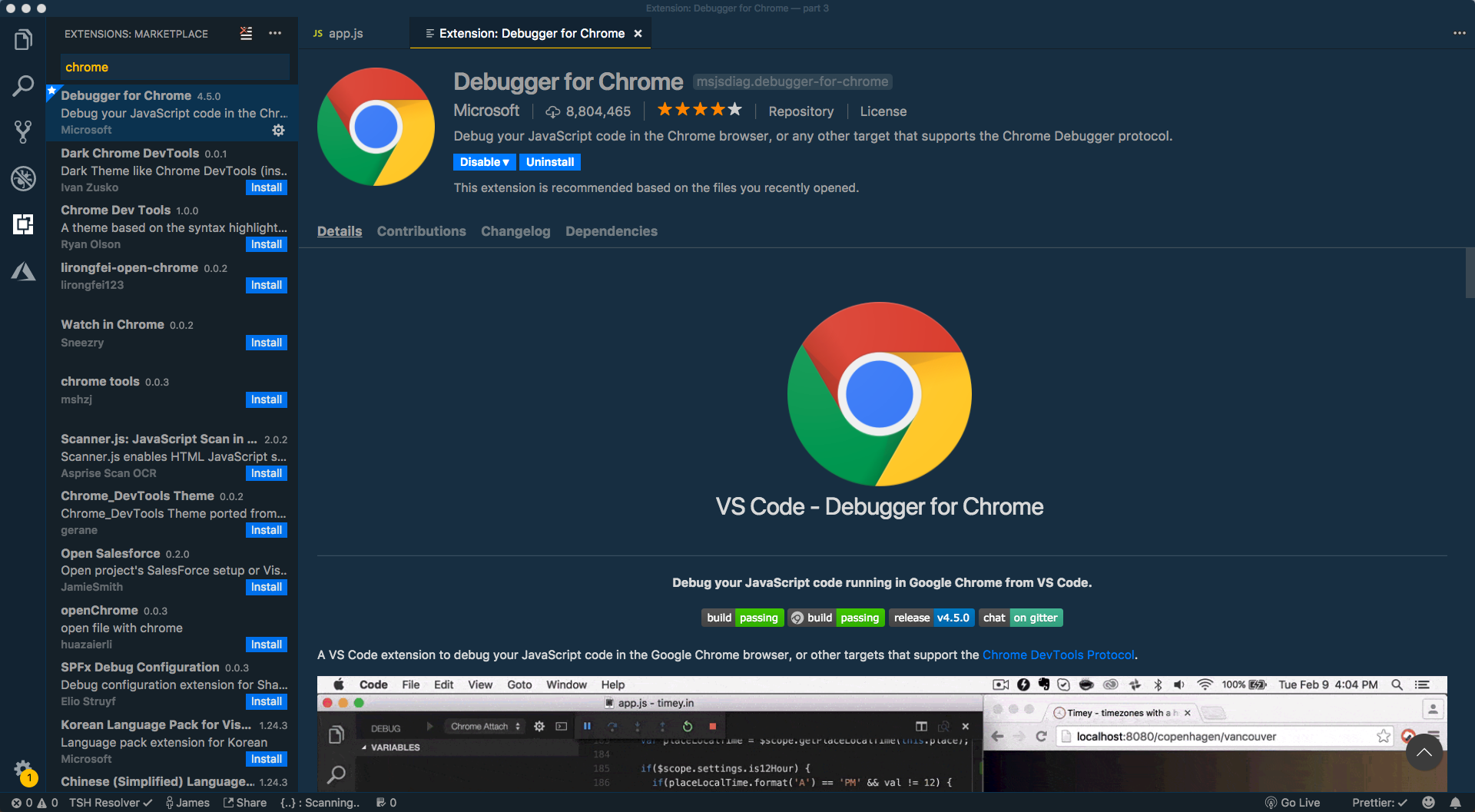
Before we can start our Node.js project we’ll need a place to write our code.
If you already have a code writing tool of choice, you can stick with it for developing your Node.js application. If you're looking for something new, we recommend trying out a few options:
- Sublime Text is a text editor popular for its ease of use and extensibility. Start here if you’re eager to get coding and don’t think you’ll want a lot of frills in your development environment.
- Visual Studio Code is an Integrated Development Environment (IDE) we like to use for JavaScript. It takes longer to set up but comes with more helpful tools already installed.
- Node.js Tools for Visual Studio is a great option if you're already a Visual Studio user.
- Vim is a perennial favorite text editor among advanced users.
If you’re new to programming, we recommend giving Sublime Text and Visual Studio Code each a try before you settle on your favorite. Many developers here at Twilio are using either - or both!
Start a New Node.js Project with 'npm init'
Before starting any new Node.js project we should run npm init
to create a new package.json file for our project.
Create a new empty directory in your development environment and run npm init
. You'll then answer a few basic questions about your project, and npm will create a new package.json file for you when you're done.
Now we're ready to install our Node.js dependencies.
Install Express.js and the Twilio Node.js SDK
We’re almost ready to start writing our Express web application, but first we need to install the Express package using npm
.
Node.js uses npm to manage dependencies, so the command to pull Express and the Twilio SDK into our development environment is npm install --save express twilio
.
The --save
flag tells npm to add the Express and Twilio packages to the dependencies
object in our project's package.json file. When we want to install these same packages again in the future - like on a production server - we can just run npm install
.
Create a Simple Express.js Application
We can test that our development environment is configured correctly by creating a simple Express application. We’ll grab the ten-line example from Express's documentation and drop it in a new file called index.js.
We can then try running our new Express application with the command node index.js
. If you open http://localhost:3000 in your browser and you should see the “Hello World!” response.
Note: If you’re using a virtual machine for your development environment, like vagrant, you might not be able to see your Express application at the localhost host name. Continue on to the ngrok section for an easy way to fix this.
Install ngrok for Local Development
Once you see your sample Express application’s “Hello World!” message, your development environment is ready to go. But for most Twilio projects you’ll want to install one more helpful tool: ngrok.

Most Twilio services use webhooks to communicate with your application. When Twilio receives an incoming phone call, for example, it reaches out to a URL in your application for instructions on how to handle the call.
When you’re working on your Express application in your development environment your app is only reachable by other programs on the same computer, so Twilio won’t be able to talk to it.
Node Js Command Prompt In Visual Studio Code
Ngrok is our favorite tool for solving this problem. Once started, it provides a unique URL on the ngrok.io domain which will forward incoming requests to your local development environment.
Visual Studio Code Install Node
To start, head over to the ngrok download page and grab the binary for your operating system: https://ngrok.com/download
Once downloaded, make sure your Express application is running and then start ngrok using this command: './ngrok http 3000'. You should see output similar to this:
Look at the “Forwarding” line to see your unique Ngrok domain name (ours is 'aaf29606.ngrok.io') and then point your browser at that domain name.
If everything’s working correctly, you should see your Express application’s “Hello World!” message displayed at your new ngrok URL.
Anytime you’re working on your Twilio application and need a URL for a webhook you should use ngrok to get a publicly accessible URL like this one.
Where to Next with Express and Node?
You’re now ready to build out your Express application! Here are a few other sample applications we've built:
More Node.js and Express Resources and Guides
Need some help?
C In Visual Studio
We all do sometimes; code is hard. Get help now from our support team, or lean on the wisdom of the crowd browsing the Twilio tag on Stack Overflow.
