. Only use optimizations that obfuscate the code if you need to. Not all of these tips are hard and fast rules. Use your judgement to determine what improvements are appropriate for your code. Be aware of QuerySet's lazy evaluation. When discussing Django ORM optimization tips recently, I realized there wasn't really a quick reference guide for them, so I decided to make one including the tips I find most helpful. You can find the cheat sheet gist here. I also wrote a slightly more in depth blog post about it here, if you're interested. I hope this is helpful to someone. Django ORM optimization cheat sheet. GitHub Gist: instantly share code, notes, and snippets.
Information gather from original post at:
https://gist.github.com/levidyrek/6db1cf88b953f3f006bf678a0f09da8e#file-django_orm_optimization_cheat_sheet-py

Caveats:
- Only use optimizations that obfuscate the code if you need to.
- Not all of these tips are hard and fast rules.
- Use your judgement to determine what improvements are appropriate for your code.
1. Profile
Use these tools:
- django-debug-toolbar
- QuerySet.explain()
2. Be aware of QuerySet’s lazy evaluation.
2a. When QuerySets are evaluated
Iteration
Slicing/Indexing
Pickling (i.e. serialization)
Evaluation functions
Other
2b. When QuerySets are cached/not cached
Not Cached
Not reusing evaluated QuerySets
Slicing/indexing unevaluated QuerySets
Printing
Cached
Reusing an evaluated QuerySet
Slicing/indexing evaluated QuerySets
3. Be aware of which attributes are not cached.
Not initially retrieved/cached
Foreign-key related objects
Never cached
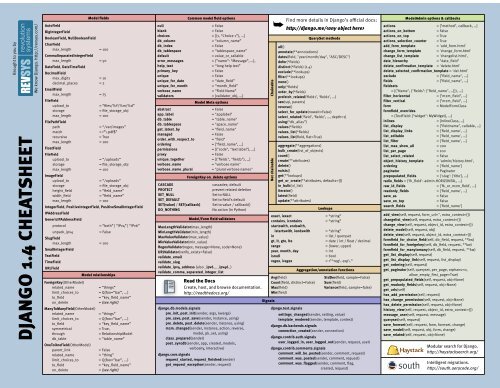
Callable attributes
4. Use select_related() and prefetch_related() when you will need everything.
DON’T
DO
5. Try to avoid database queries in a loop.
DON’T (contrived example)
DO (contrived example)
6. Use iterator() to iterate through a very large QuerySet only once.
Save memory by not caching anything
7. Do work in the database rather than in Python.

7a. Use filter() and exclude()
DON’T
DO
7b. Use F expressions
Django Orm Cheat Sheet Free

DON’T
DO
7c. Do aggregation in the database, if possible
DON’T
DO
8. Use values() and values_list() to get only the things you need.
8a. Use values()
DON’T
DO
8b. Use values_list()
DON’T
DO
- Use defer() and only() when you know you won’t need certain fields.
- Use when you need a QuerySet instead of a list of dicts from values().
- Really only useful to defer fields that require significant processing to convert to a python object.
9a. Use defer()
9b. Use only()
- Use count() and exists() when you don’t need the contents of the QuerySet.
* Caveat: Only use these when you don’t need to evaluate the QuerySet.
10a. Use count()
DON’T
DO
10b. Use exists()
DON’T
Django Orm Cheat Sheet Pdf
DO
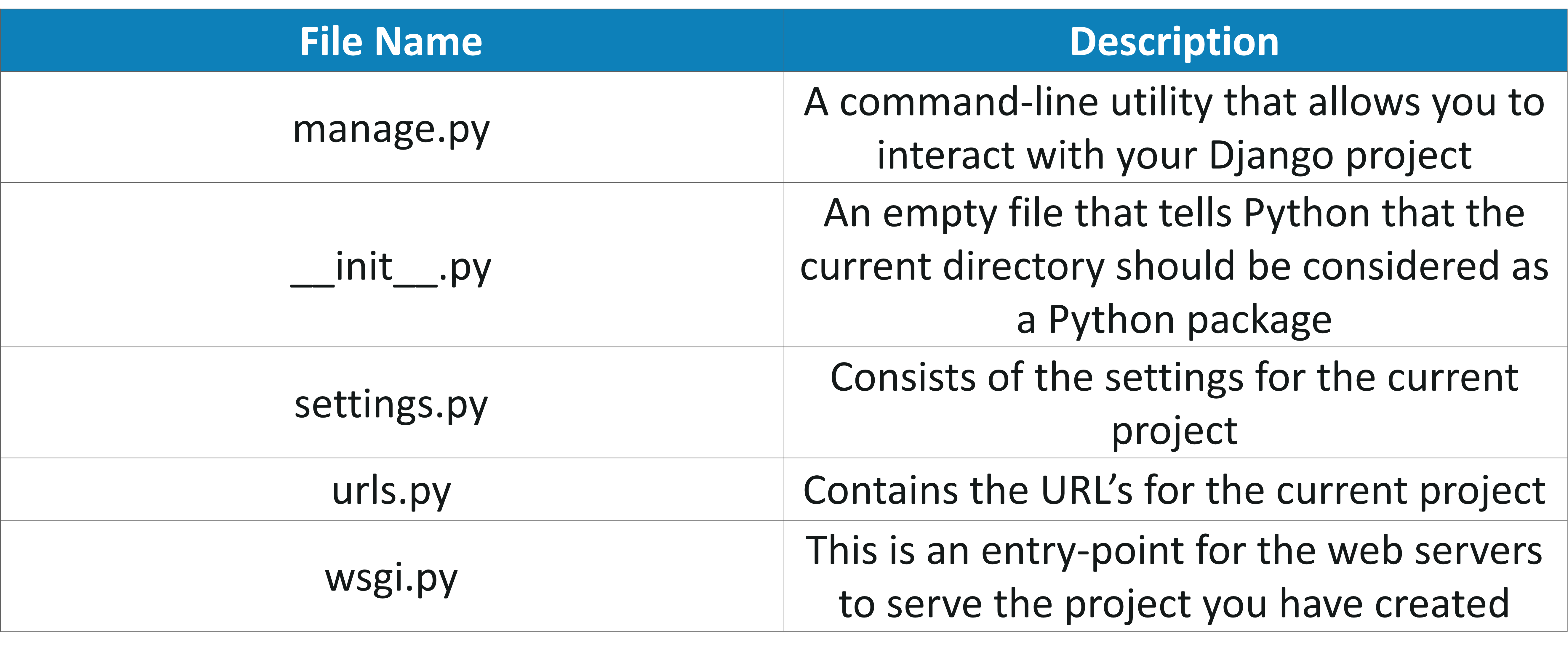
11. Use delete() and update() when possible.
Django Orm Cheat Sheet Printable
11a. Use delete()
DON’T
DO
11b. Use update()
DON’T
DO
- Use bulk_create() when possible.
* Caveats: https://docs.djangoproject.com/en/2.1/ref/models/querysets/#django.db.models.query.QuerySet.bulk_create
Bulk Create
Bulk add to many-to-many fields
13. Use foreign key values directly.
DON’T
DO
